Introduction
The same <my-counter/>
Web Component has been written in 61 variants. The complete collection is available in the WebComponents.dev Studio Editor.
In this new release, we fixed performance computation support for librairies missing from the previous editions: you can now compare both sizes and performances for all of the 61 variants! We're also pleased to welcome the 60th variant with node-projects/base-custom-webcomponent
.
Update 02/18/2022: We forgot to include one newcomer to this benchmark in our last release. So, welcome to html-element-property-mixins
which allow us to pass the bar of 60 variants! It's also a very nice challenger, arriving in all top-tens with a lightweight and performant approach that helps to bring reactivity in Web Components. Well done!
We made the choice of building a simple component like the counter for the sake of simplicity. This choice is debatable especially when it comes to comparing a bundled set of a same component vs a real application made of different components, but it produces a nice overview.
Table of Content
Source code and Bundle analysis
Select implementation:
HTMLElement
Homepage: https://html.spec.whatwg.org/multipage/custom-elements.html
Try in WebComponents.devconst template = document.createElement('template');
template.innerHTML = `
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button id="dec">-</button>
<span id="count"></span>
<button id="inc">+</button>`;
class MyCounter extends HTMLElement {
constructor() {
super();
this.count = 0;
this.attachShadow({ mode: 'open' });
}
connectedCallback() {
this.shadowRoot.appendChild(template.content.cloneNode(true));
this.shadowRoot.getElementById('inc').onclick = () => this.inc();
this.shadowRoot.getElementById('dec').onclick = () => this.dec();
this.update(this.count);
}
inc() {
this.update(++this.count);
}
dec() {
this.update(--this.count);
}
update(count) {
this.shadowRoot.getElementById('count').innerHTML = count;
}
}
customElements.define('my-counter', MyCounter);
No dependencies
Component size including library
Minified - Uncompressed | 1,009 |
Minified + Gzip | 512 |
Minified + Brotli | 367 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
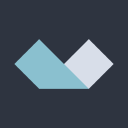
HTMLElement (w/ Alpine JS)
A rugged, minimal framework for composing JavaScript behavior in your markup.
Homepage: https://github.com/alpinejs/alpine
GitHub: https://github.com/alpinejs/alpine
Try in WebComponents.devimport Alpine from 'alpinejs'
const template = document.createElement("template");
template.innerHTML = `
<style>
span, button {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<div x-data="$el.parentElement.data()">
<button @click="dec()">-</button>
<span x-text="count"></span>
<button @click="inc()">+</button>
</div>
`;
export class MyCounter extends HTMLElement {
connectedCallback() {
this.append(template.content.cloneNode(true));
}
data() {
return {
count: 0,
inc() {
this.count++;
},
dec() {
this.count--;
},
};
}
}
customElements.define("my-counter", MyCounter);
Alpine.start();
Dependencies
alpine :
Component size including library
Minified - Uncompressed | 49,316 |
Minified + Gzip | 18,315 |
Minified + Brotli | 16,483 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
HTMLElement (w/ catalyst)
Catalyst is a set of patterns and techniques for developing components within a complex application. At its core, Catalyst simply provides a small library of functions to make developing Web Components easier.
Homepage: https://github.github.io/catalyst/
GitHub: https://github.com/github/catalyst
Try in WebComponents.devimport { controller, target } from "@github/catalyst"
const template = document.createElement("template");
template.innerHTML = `<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button data-action="click:my-counter#dec">-</button>
<span data-target="my-counter.output"></span>
<button data-action="click:my-counter#inc">+</button>
`;
@controller
class MyCounterElement extends HTMLElement {
@target output: HTMLElement;
count: number = 0;
connectedCallback() {
this.attachShadow({ mode: "open" });
this.shadowRoot.appendChild(template.content.cloneNode(true));
this.update();
}
inc() {
this.count++;
this.update();
}
dec() {
this.count--;
this.update();
}
update() {
this.output.textContent = "" + this.count;
}
}
Dependencies
@github/catalyst : ^1.2.1
Component size including library
Minified - Uncompressed | 4,732 |
Minified + Gzip | 1,922 |
Minified + Brotli | 1,656 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
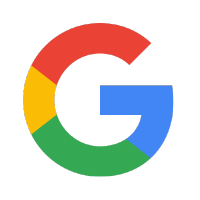
HTMLElement (w/ inc.-dom)
Homepage: http://google.github.io/incremental-dom/
GitHub: https://github.com/google/incremental-dom
Try in WebComponents.devimport { elementOpen, elementClose, text, patch } from "incremental-dom";
class MyCounter extends HTMLElement {
constructor() {
super();
this.count = 0;
this.attachShadow({ mode: "open" });
}
get styles() {
return `
span,button {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
}
connectedCallback() {
this.update();
}
inc() {
this.count++;
this.update();
}
dec() {
this.count--;
this.update();
}
update() {
patch(this.shadowRoot, () => {
elementOpen("style");
text(this.styles);
elementClose("style");
elementOpen("button", null, ["onclick", this.dec.bind(this)]);
text("-");
elementClose("button");
elementOpen("span");
text(this.count);
elementClose("span");
elementOpen("button", null, ["onclick", this.inc.bind(this)]);
text("+");
elementClose("button");
});
}
}
customElements.define("my-counter", MyCounter);
Dependencies
incremental-dom : ^0.7.0
Component size including library
Minified - Uncompressed | 7,605 |
Minified + Gzip | 3,153 |
Minified + Brotli | 2,805 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
HTMLElement (w/ jtml)
@github/jtml - This library is designed as a layer on top of @github/template-parts to provide declarative, JavaScript based HTML template tags.
Homepage: https://www.npmjs.com/package/@github/jtml
Try in WebComponents.devimport { html, render } from "@github/jtml";
class MyCounter extends HTMLElement {
constructor() {
super();
this.count = 0;
}
connectedCallback() {
this.attachShadow({mode: 'open'})
this.update()
}
inc() {
this.count++;
this.update();
}
dec() {
this.count--;
this.update();
}
update() {
render(html`
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button onclick="${() => this.dec()}">-</button>
<span>${this.count}</span>
<button onclick="${() => this.inc()}">+</button>
`,
this.shadowRoot);
}
}
customElements.define("my-counter", MyCounter);
Dependencies
@github/jtml : ^0.4.1
Component size including library
Minified - Uncompressed | 8,797 |
Minified + Gzip | 2,675 |
Minified + Brotli | 2,338 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
HTMLElement (w/ Lighterhtml)
The hyperHTML strength & experience without its complexity
Homepage: https://medium.com/@WebReflection/lit-html-vs-hyperhtml-vs-lighterhtml-c084abfe1285
GitHub: https://github.com/WebReflection/lighterhtml
Try in WebComponents.devimport { html, render } from 'lighterhtml';
class MyCounter extends HTMLElement {
constructor() {
super();
this.count = 0;
this.attachShadow({ mode: 'open' });
}
connectedCallback() {
this.update();
}
inc = () => {
this.count++;
this.update();
};
dec = () => {
this.count--;
this.update();
};
style() {
return `
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`;
}
template() {
return html`
<style>
${this.style()}
</style>
<button onclick="${this.dec}">-</button>
<span>${this.count}</span>
<button onclick="${this.inc}">+</button>
`;
}
update() {
render(this.shadowRoot, this.template());
}
}
customElements.define('my-counter', MyCounter);
Dependencies
lighterhtml : ^4.2.0
Component size including library
Minified - Uncompressed | 12,231 |
Minified + Gzip | 5,291 |
Minified + Brotli | 4,794 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
HTMLElement (w/ Lit 2.0)
Lit - Simple. Fast. Web Components.
Homepage: https://lit.dev
GitHub: https://github.com/lit/lit
Try in WebComponents.devimport { html, render } from 'lit';
class MyCounter extends HTMLElement {
constructor() {
super();
this.count = 0;
this.attachShadow({ mode: 'open' });
}
connectedCallback() {
this.update();
}
inc() {
this.count++;
this.update();
}
dec() {
this.count--;
this.update();
}
style() {
return /*css*/ `
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`;
}
template() {
return html`
<style>
${this.style()}
</style>
<button @click="${this.dec}">-</button>
<span>${this.count}</span>
<button @click="${this.inc}">+</button>
`;
}
update() {
render(this.template(), this.shadowRoot, { host: this });
}
}
customElements.define('my-counter', MyCounter);
Dependencies
lit : ^2.1.3
Component size including library
Minified - Uncompressed | 16,260 |
Minified + Gzip | 5,852 |
Minified + Brotli | 5,297 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
HTMLElement (w/ Lit-html)
Lit-Html - An efficient, expressive, extensible HTML templating library for JavaScript
Homepage: https://lit-html.polymer-project.org/
GitHub: https://github.com/polymer/lit-html
Try in WebComponents.devimport { html, render } from 'lit-html';
class MyCounter extends HTMLElement {
constructor() {
super();
this.count = 0;
this.attachShadow({ mode: 'open' });
}
connectedCallback() {
this.update();
}
inc() {
this.count++;
this.update();
}
dec() {
this.count--;
this.update();
}
style() {
return `
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`;
}
template() {
return html`
<style>
${this.style()}
</style>
<button @click="${this.dec}">-</button>
<span>${this.count}</span>
<button @click="${this.inc}">+</button>
`;
}
update() {
render(this.template(), this.shadowRoot, { host: this });
}
}
customElements.define('my-counter', MyCounter);
Dependencies
lit-html : ^2.1.3
Component size including library
Minified - Uncompressed | 8,705 |
Minified + Gzip | 3,579 |
Minified + Brotli | 3,220 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
HTMLElement (w/ Mithril)
A JavaScript Framework for Building Brilliant Applications
Homepage: https://mithril.js.org/
GitHub: https://github.com/MithrilJS/mithril.js
Try in WebComponents.dev/* @jsx m */
import m from "mithril";
export class MyCounter extends HTMLElement {
count: number = 0;
constructor() {
super();
this.attachShadow({ mode: "open" });
}
get styles() {
return (
<style>{`
span,button {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`}</style>
);
}
inc() {
this.count++;
this.update();
}
dec() {
this.count--;
this.update();
}
connectedCallback() {
this.update();
}
update() {
m.render(
this.shadowRoot as any,
<div>
{this.styles}
<button onclick={this.dec.bind(this)}>-</button>
<span>{this.count}</span>
<button onclick={this.inc.bind(this)}>+</button>
</div>
);
}
}
customElements.define("my-counter", MyCounter);
Dependencies
mithril : ^2.0.4
Component size including library
Minified - Uncompressed | 28,361 |
Minified + Gzip | 10,167 |
Minified + Brotli | 9,100 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
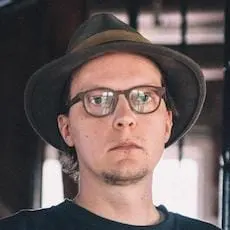
HTMLElement (w/ Sidewind)
Sidewind - Tailwind but for state
Homepage: https://sidewind.js.org/
GitHub: https://github.com/survivejs/sidewind
Try in WebComponents.devimport 'sidewind';
const template = document.createElement("template");
template.innerHTML = `
<style>
span, button {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<div x-state="0">
<button onclick="setState(counter => counter - 1)"> - </button>
<span x="state"></span>
<button onclick="setState(counter=> counter + 1)"> + </button>
</div>
`;
export class MyCounter extends HTMLElement {
connectedCallback() {
this.append(template.content.cloneNode(true));
evaluateAllDirectives()
}
}
customElements.define("my-counter", MyCounter);
Dependencies
sidewind : ^6.0.2
Component size including library
Minified - Uncompressed | 7,580 |
Minified + Gzip | 2,887 |
Minified + Brotli | 2,535 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
HTMLElement (w/ uhtml)
micro html is a ~2.5K lighterhtml subset to build declarative and reactive UI via template literals tags.
Homepage: https://github.com/WebReflection/uhtml
GitHub: https://github.com/WebReflection/uhtml
Try in WebComponents.devimport { html, render } from "uhtml";
class MyCounter extends HTMLElement {
constructor() {
super();
this.count = 0;
this.attachShadow({ mode: "open" });
}
connectedCallback() {
this.update();
}
inc = () => {
this.count++;
this.update();
};
dec = () => {
this.count--;
this.update();
};
template() {
return html`
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button onclick="${this.dec}">-</button>
<span>${this.count}</span>
<button onclick="${this.inc}">+</button>
`;
}
update() {
render(this.shadowRoot, this.template());
}
}
customElements.define("my-counter", MyCounter);
Dependencies
uhtml : ^2.8.0
Component size including library
Minified - Uncompressed | 7,434 |
Minified + Gzip | 3,361 |
Minified + Brotli | 3,024 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
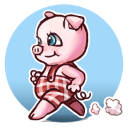
AppRun
AppRun is a JavaScript library for building reliable, high-performance web applications using the Elm inspired architecture, events, and components.
Homepage: https://apprun.js.org/
GitHub: https://github.com/yysun/apprun
Try in WebComponents.dev/** @jsx app.h */
import { app, Component } from "apprun";
// import { html } from 'lit-html';
const styles = `.my-counter * {
font-size: 200%;
}
.my-counter span {
width: 4rem;
display: inline-block;
text-align: center;
}
.my-counter button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
const add = (state, num) => state + num;
export class MyCounter extends Component {
state = 0;
inc = state => state + 1;
dec = state => state - 1;
view = state => <div class="my-counter">
<style>{styles}</style>
<button $onclick={this.dec}>-</button>
<span>{state}</span>
<button $onclick={this.inc}>+</button>
</div>
}
app.webComponent('my-counter', MyCounter);
Dependencies
apprun : ^3.28.8
Component size including library
Minified - Uncompressed | 15,564 |
Minified + Gzip | 5,383 |
Minified + Brotli | 4,806 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
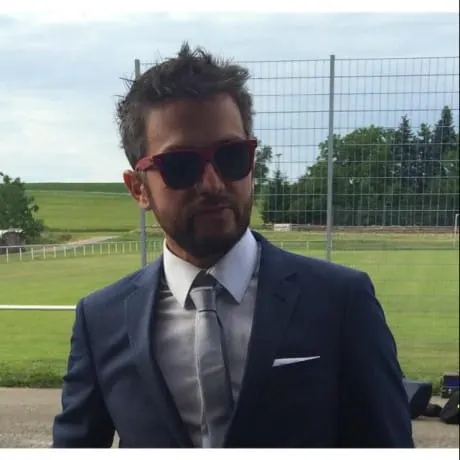
Base Custom Webcomponent
The base-custom-webcomponent is a simple base class for the use of webcomponents in typescript.
Homepage: https://github.com/node-projects/base-custom-webcomponent
GitHub: https://github.com/node-projects/base-custom-webcomponent
Try in WebComponents.devimport { html, css, BaseCustomWebComponentLazyAppend } from "@node-projects/base-custom-webcomponent";
class MyCounter extends BaseCustomWebComponentLazyAppend {
static style = css`
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
static template = html`
<button @click="[[this.dec()]]">-</button>
[[this.count]]
<button @click="[[this.inc()]]">+</button>`;
count = 0;
constructor() {
super();
this._bindingsParse();
}
inc() {
this.count++;
this._bindingsRefresh();
}
dec() {
this.count--;
this._bindingsRefresh();
}
}
customElements.define('my-counter', MyCounter);
Dependencies
@node-projects/base-custom-webcomponent : ^0.5.3
Component size including library
Minified - Uncompressed | 11,653 |
Minified + Gzip | 3,475 |
Minified + Brotli | 3,048 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Compiled and/or Transpiled by WebComponents.dev compiler.Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
CanJS
Build CRUD apps in fewer lines of code.
Homepage: https://canjs.com/
GitHub: https://github.com/canjs/canjs
Try in WebComponents.devimport { StacheElement } from 'can/core';
class MyCounter extends StacheElement {
constructor() {
super();
this.viewRoot = this.attachShadow({ mode: 'open' });
}
static view = `<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button on:click="this.decrement()">-</button>
<span>{{ this.count }}</span>
<button on:click="this.increment()">+</button>`;
static props = {
count: 0,
};
increment() {
this.count++;
}
decrement() {
this.count--;
}
}
customElements.define('my-counter', MyCounter);
Dependencies
can : ^6.6.2
Component size including library
Minified - Uncompressed | 347,803 |
Minified + Gzip | 97,639 |
Minified + Brotli | 83,527 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
ElemX
Library for connecting MobX to native Web Components with a Vue-like template binding syntax
Homepage: https://github.com/agquick/elemx.js
GitHub: https://github.com/agquick/elemx.js
Try in WebComponents.devimport { observable } from "mobx";
import { ReactiveElement } from "elemx";
class MyCounterElement extends ReactiveElement {
@observable counter = 0;
inc() {
this.counter += 1;
}
dec() {
this.counter -= 1;
}
templateHTML() {
return `
<button @click="this.dec()">-</button>
{{ this.counter }}
<button @click="this.inc()">+</button>
`;
}
templateCSS() {
return `
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`;
}
}
customElements.define('my-counter', MyCounterElement);
Dependencies
elemx : ^0.2.2
mobx : ^5.15.4
Component size including library
Minified - Uncompressed | 53,017 |
Minified + Gzip | 15,735 |
Minified + Brotli | 14,047 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Exalt
A JavaScript framework for building universal apps.
GitHub: https://github.com/exalt/exalt
Try in WebComponents.devimport { Component, html } from "@exalt/core";
import { define } from "@exalt/core/decorators";
const style = /*css*/`
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`;
@define({ tag: "my-counter", styles: [style] })
export class MyCounter extends Component {
counter = super.reactive(0);
render() {
return html`
<button onclick=${() => this.counter--}>-</button>
<span>${this.counter}</span>
<button onclick=${() => this.counter++}>+</button>
`;
}
}
Dependencies
@exalt/core : ^1.1.5
Component size including library
Minified - Uncompressed | 6,854 |
Minified + Gzip | 2,804 |
Minified + Brotli | 2,474 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
FAST
The adaptive interface system for modern web experiences
Homepage: https://www.fast.design/
GitHub: https://github.com/microsoft/fast
Try in WebComponents.devimport { html, css, FASTElement } from "@microsoft/fast-element";
class MyCounter extends FASTElement {
static definition = {
name: "my-counter",
template: html<MyCounter>`
<button @click="${(x) => x.count--}">-</button>
<span>${(x) => x.count}</span>
<button @click="${(x) => x.count++}">+</button>
`,
styles: css`
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`,
attributes: ["count"],
};
count: number = 0;
}
FASTElement.define(MyCounter);
Dependencies
@microsoft/fast-element : ^1.7.0
Component size including library
Minified - Uncompressed | 25,496 |
Minified + Gzip | 7,792 |
Minified + Brotli | 6,991 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Compiled and/or Transpiled by WebComponents.dev compiler.Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
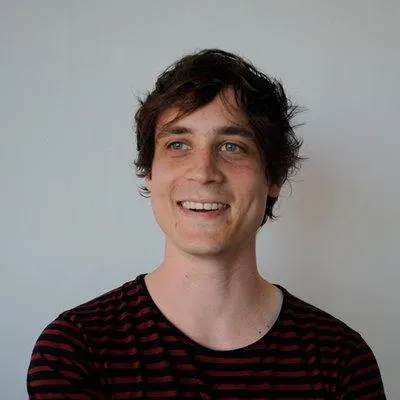
html-element-property-mixins
html-element-property-mixins is a collection of mixins extending HTMLElement with properties, powering custom elements.
Homepage: https://github.com/woutervroege/html-element-property-mixins
GitHub: https://github.com/woutervroege/html-element-property-mixins
Try in WebComponents.devimport { ObservedProperties } from 'html-element-property-mixins';
class MyCounter extends ObservedProperties(HTMLElement) {
static get observedProperties() {
return ['count'];
}
static get template() {
return /*html*/ `
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button id="dec">-</button>
<span id="count">0</span>
<button id="inc">+</button>
`
}
constructor() {
super();
this.count = 0;
this.attachShadow({ mode: 'open' });
this.shadowRoot.innerHTML = this.constructor.template;
this.shadowRoot.getElementById('inc').onclick = () => this.count++;
this.shadowRoot.getElementById('dec').onclick = () => this.count--;
}
propertyChangedCallback() {
this.update();
}
update() {
if(!this.shadowRoot) return;
this.shadowRoot.getElementById('count').innerHTML = this.count;
}
}
customElements.define('my-counter', MyCounter);
Dependencies
html-element-property-mixins : ^0.11.0
Component size including library
Minified - Uncompressed | 2,596 |
Minified + Gzip | 912 |
Minified + Brotli | 740 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
HyperHTML Element
Framework agnostic, hyperHTML can be used to render any view, including Custom Elements and Web Components.
GitHub: https://github.com/WebReflection/hyperHTML-Element
Try in WebComponents.devimport HyperHTMLElement from "hyperhtml-element";
class MyCounter extends HyperHTMLElement {
constructor() {
super();
this.attachShadow({ mode: "open" });
}
created() {
this.count = 0;
this.render();
}
inc = () => {
this.count++;
this.render();
};
dec = () => {
this.count--;
this.render();
};
render() {
return this.html`
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button onclick=${this.dec}>-</button>
<span>${this.count}</span>
<button onclick=${this.inc}>+</button>
`;
}
}
MyCounter.define("my-counter");
Dependencies
hyperhtml-element : ^3.15.2
Component size including library
Minified - Uncompressed | 23,131 |
Minified + Gzip | 9,201 |
Minified + Brotli | 8,384 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
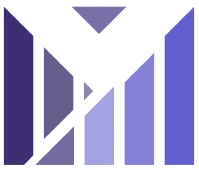
Joist
A small (~2kb) library to help with the creation of web components and web component based applications
Homepage: https://github.com/joist-framework/joist
GitHub: https://github.com/joist-framework/joist
Try in WebComponents.dev// By Danny Blue GitHub:deebloo
import { component, JoistElement, handle, get, State } from "@joist/component";
@component({
tagName: "my-counter",
shadowDom: "open",
styles: [
`* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`,
],
state: 0,
render: ({ state, host, run }) => {
const self = host.shadowRoot;
if (!self.innerHTML) {
self.innerHTML = `
<button id="dec">-</button>
<span id="count"></span>
<button id="inc">+</button>`;
self.getElementById("inc").addEventListener("click", run("add", 1));
self.getElementById("dec").addEventListener("click", run("add", -1));
}
self.getElementById("count").innerHTML = state.toString();
},
})
export class CounterElement extends JoistElement {
@get(State) private state!: State<number>;
@handle("add") add(_: Event, value: number) {
this.state.setValue(this.state.value + value);
}
}
Dependencies
@joist/component : ^1.8.8
Component size including library
Minified - Uncompressed | 6,218 |
Minified + Gzip | 2,273 |
Minified + Brotli | 2,003 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
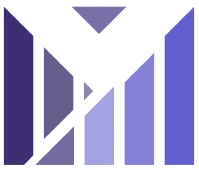
Joist (with lit-html)
A small (~2kb) library to help with the creation of web components and web component based applications
Homepage: https://github.com/joist-framework/joist
GitHub: https://github.com/joist-framework/joist
Try in WebComponents.dev// By Danny Blue GitHub:deebloo
import { component, JoistElement, handle, get, State } from "@joist/component";
import { template } from "@joist/component/lit-html";
import { html } from "lit-html";
@component({
tagName: "my-counter",
shadowDom: "open",
state: 0,
styles: [
`* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`,
],
render: template(({ state, run }) => {
return html`
<button @click="${run("update", -1)}">-</button>
<span>${state}</span>
<button @click="${run("update", 1)}">+</button>
`;
}),
})
export class CounterElement extends JoistElement {
@get(State) private state!: State<number>;
@handle("update")
updateCount(_: Event, num: number) {
return this.state.setValue(this.state.value + num);
}
}
Dependencies
@joist/component : ^1.8.8
lit-html : ^2.1.3
Component size including library
Minified - Uncompressed | 13,888 |
Minified + Gzip | 5,213 |
Minified + Brotli | 4,733 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Lit 2.0 JavaScript
Lit - Simple. Fast. Web Components.
Homepage: https://lit.dev/
GitHub: https://github.com/lit/lit
Try in WebComponents.devimport { LitElement, html, css } from "lit";
export class MyCounter extends LitElement {
static properties = {
count: { type: Number },
};
static styles = css`
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`;
constructor() {
super();
this.count = 0;
}
inc() {
this.count++;
}
dec() {
this.count--;
}
render() {
return html`
<button @click="${this.dec}">-</button>
<span>${this.count}</span>
<button @click="${this.inc}">+</button>
`;
}
}
customElements.define("my-counter", MyCounter);
Dependencies
lit : ^2.1.3
Component size including library
Minified - Uncompressed | 16,397 |
Minified + Gzip | 5,981 |
Minified + Brotli | 5,405 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Lit 2.0 TypeScript
Lit - Simple. Fast. Web Components.
Homepage: https://lit.dev/
GitHub: https://github.com/lit/lit
Try in WebComponents.devimport {html, css, LitElement} from 'lit';
import {customElement, property} from 'lit/decorators.js';
@customElement('my-counter')
export class SimpleGreeting extends LitElement {
@property() public count = 0;
static styles = css`
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
render() {
return html`
<button @click="${this.dec}">-</button>
<span>${this.count}</span>
<button @click="${this.inc}">+</button>
`;
}
inc() {
this.count++;
}
dec() {
this.count--;
}
}
Dependencies
lit : ^2.1.3
Component size including library
Minified - Uncompressed | 17,576 |
Minified + Gzip | 6,409 |
Minified + Brotli | 5,768 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Compiled and/or Transpiled by WebComponents.dev compiler.Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
LitElement v2
Homepage: https://lit-element.polymer-project.org/
GitHub: https://github.com/polymer/lit-element
Try in WebComponents.devimport { LitElement, html, css } from 'lit-element';
export class MyCounter extends LitElement {
static properties = {
count: { type: Number },
};
static styles = css`
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`;
constructor() {
super();
this.count = 0;
}
inc() {
this.count++;
}
dec() {
this.count--;
}
render() {
return html`
<button @click="${this.dec}">-</button>
<span>${this.count}</span>
<button @click="${this.inc}">+</button>
`;
}
}
customElements.define('my-counter', MyCounter);
Dependencies
lit-element : ^3.1.2
Component size including library
Minified - Uncompressed | 16,748 |
Minified + Gzip | 6,170 |
Minified + Brotli | 5,567 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
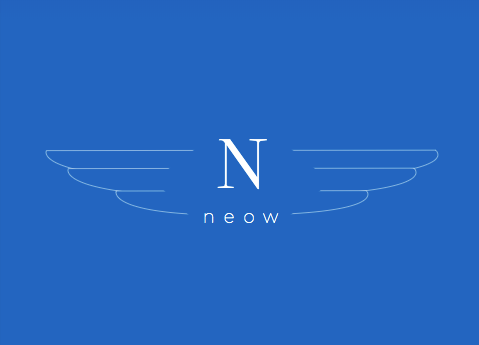
Neow (Alpha)
Modern, fast, and light it's the front-end framework to fall inlove with. (ALPHA)
Homepage: https://neow.dev
GitHub: (alpha)
Try in WebComponents.devimport { ComponentMixin } from "@neow/core";
class MyComponent extends ComponentMixin(HTMLElement) {
static template = `
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button onclick="{{this.dec()}}">-</button>
<span>{{this.counter}}</span>
<button onclick="{{this.inc()}}">+</button>
`;
counter = 0;
inc() {
this.counter++;
}
dec() {
this.counter--;
}
}
customElements.define("my-counter", MyComponent);
Dependencies
@neow/core : ^1.0.1
Component size including library
Minified - Uncompressed | 4,445 |
Minified + Gzip | 2,001 |
Minified + Brotli | 1,727 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
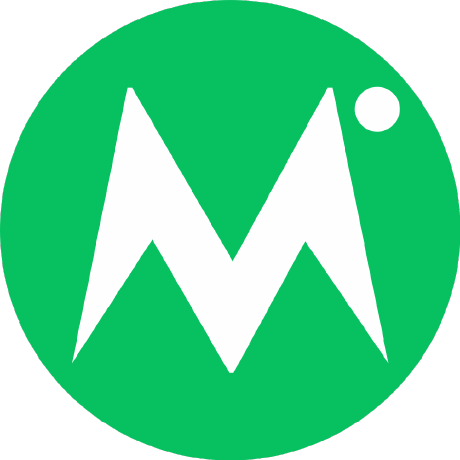
Omi w/Class
Front End Cross-Frameworks Framework - Merge Web Components, JSX, Virtual DOM, Functional style, observe or Proxy into one framework with tiny size and high performance. Write components once, using in everywhere, such as Omi, React, Preact, Vue or Angular.
Homepage: http://omijs.org
GitHub: https://github.com/Tencent/omi
Try in WebComponents.dev/** @jsx h */
import { WeElement, define, h } from "omi";
define(
"my-counter",
class extends WeElement {
data = {
count: 0,
};
static css = `
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
dec = () => {
this.data.count--;
this.update();
};
inc = () => {
this.data.count++;
this.update();
};
render() {
return (
<h.f>
<button onClick={this.dec}>-</button>
<span>{this.data.count}</span>
<button onClick={this.inc}>+</button>
</h.f>
);
}
}
);
Dependencies
omi : ^6.23.0
Component size including library
Minified - Uncompressed | 20,841 |
Minified + Gzip | 7,233 |
Minified + Brotli | 6,511 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
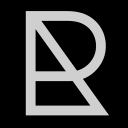
Readymade
JavaScript microlibrary for developing Web Components with Decorators
Homepage: https://github.com/readymade-ui/readymade
GitHub: https://github.com/readymade-ui/readymade
Try in WebComponents.devimport { Component, CustomElement, html, css } from '@readymade/core';
@Component({
selector: 'my-counter',
template: html`
<button id="dec">-</button>
<span id="count">0</span>
<button id="inc">+</button>
`,
style: css`
span,
button {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`
})
export class MyCounter extends CustomElement {
private $state: number = 0;
constructor() {
super();
}
connectedCallback() {
this.shadowRoot
.querySelector('#inc')
.addEventListener('click', this.inc.bind(this));
this.shadowRoot
.querySelector('#dec')
.addEventListener('click', this.dec.bind(this));
}
get count(): number {
return this.$state;
}
set count(count: number) {
this.$state = count;
}
inc() {
this.count = this.count + 1;
this.update();
}
dec() {
this.count = this.count - 1;
this.update();
}
update() {
const print = this.count.toString();
(this.shadowRoot.querySelector('#count') as HTMLSpanElement).innerText = print;
}
}
Dependencies
@readymade/core : ^2.0.1
Component size including library
Minified - Uncompressed | 2,553 |
Minified + Gzip | 1,148 |
Minified + Brotli | 923 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
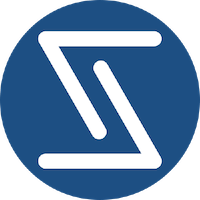
SkateJS (with Lit-html)
SkateJS - Effortless custom elements powered by modern view libraries.
Homepage: https://skatejs.netlify.com/
GitHub: https://github.com/skatejs/skatejs
Try in WebComponents.devimport Element from "@skatejs/element";
import { render, html } from "lit-html";
class MyCounterElement extends Element {
static get props() {
return {
count: Number,
};
}
inc = () => {
this.count++;
};
dec = () => {
this.count--;
};
render() {
const style = `
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
return html`
<style>
${style}
</style>
<button @click="${this.dec}">
-
</button>
<span>${this.count}</span>
<button @click="${this.inc}">
+
</button>
`;
}
renderer() {
return render(this.render(), this.renderRoot);
}
}
customElements.define("my-counter", MyCounterElement);
Dependencies
@skatejs/element : 0.0.1
lit-html : ^2.1.3
Component size including library
Minified - Uncompressed | 14,065 |
Minified + Gzip | 5,201 |
Minified + Brotli | 4,689 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
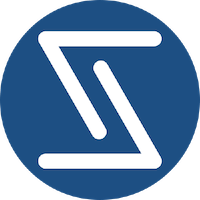
SkateJS (with Preact)
SkateJS - Effortless custom elements powered by modern view libraries.
Homepage: https://skatejs.netlify.com/
GitHub: https://github.com/skatejs/skatejs
Try in WebComponents.dev/** @jsx h **/
import Element, { h } from "@skatejs/element-preact";
class MyCounterElement extends Element {
static props = {
count: Number,
}
inc = () => {
this.count++;
};
dec = () => {
this.count--;
};
render() {
const style = `host * {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
return (
<host>
<style>{style}</style>
<button onclick={this.dec}>-</button>
<span>{this.count}</span>
<button onclick={this.inc}>+</button>
</host>
);
}
}
customElements.define("my-counter", MyCounterElement);
Dependencies
@skatejs/element-preact : 0.0.1
Component size including library
Minified - Uncompressed | 16,565 |
Minified + Gzip | 5,894 |
Minified + Brotli | 5,321 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
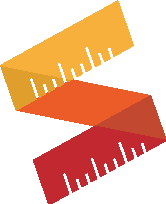
SlimJS
Slim.js is a lightning fast library for development of native Web Components and Custom Elements based on modern standards. No black magic involved, no useless dependencies.
Homepage: https://slimjs.com
GitHub: https://github.com/slimjs/slim.js
Try in WebComponents.devimport { Slim } from 'slim-js';
import { tag, template } from 'slim-js/decorators';
import 'slim-js/directives';
@tag("my-counter")
@template(`
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style >
<button @click="this.dec()">-</button>
<span>{{this.count}}</span>
<button @click="this.inc()">+</button>
`)
class MyCounter extends Slim {
count = 0;
inc () {
this.count++
}
dec () {
this.count--
}
}
Dependencies
slim-js : ^5.0.10
Component size including library
Minified - Uncompressed | 14,319 |
Minified + Gzip | 4,982 |
Minified + Brotli | 4,204 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
uce-template
A tiny toolless library with tools included
Homepage: https://medium.com/@WebReflection/a-new-web-components-wonder-f9e042785a91
GitHub: https://github.com/WebReflection/uce-template
Try in WebComponents.dev<my-counter>
<button onclick="{{dec}}">
-
</button>
<span>{{state.count}}</span>
<button onclick="{{inc}}">
+
</button>
</my-counter>
<script type="module">
import reactive from "@uce/reactive";
export default {
setup(element) {
const state = reactive({ count: 0 });
const inc = () => {
state.count++;
};
const dec = () => {
state.count--;
};
return { state, inc, dec };
},
};
</script>
<style scoped>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
Dependencies
uce-template : ^0.7.1
Component size including library
Minified - Uncompressed | 26,008 |
Minified + Gzip | 10,290 |
Minified + Brotli | 9,358 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Wafer
A simple and lightweight base library for building Web Components that can be used on the browser, server or both
Homepage: https://waferlib.netlify.app/
GitHub: https://github.com/lamplightdev/wafer
Try in WebComponents.dev/**
* Uses Wafer (https://waferlib.netlify.app/)
*/
import Wafer from "@lamplightdev/wafer";
export class MyCounter extends Wafer {
static get template() {
return `
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button id="dec">-</button>
<span id="count"></span>
<button id="inc">+</button>
`;
}
static get props() {
return {
count: {
type: Number,
initial: 0,
targets: [{
selector: '$#count',
text: true,
}]
}
}
}
get events() {
return {
'$#inc': {
click: () => this.count++
},
'$#dec': {
click: () => this.count--
}
}
}
}
customElements.define("my-counter", MyCounter);
Dependencies
@lamplightdev/wafer : ^1.0.14
Component size including library
Minified - Uncompressed | 6,422 |
Minified + Gzip | 2,356 |
Minified + Brotli | 2,077 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Atomico
Atomico is a microlibrary (3.9kB) inspired by React Hooks, designed and optimized for the creation of small, powerful, declarative webcomponents and only using functions
Homepage: https://atomico.gitbook.io/doc/
GitHub: https://github.com/atomicojs/atomico
Try in WebComponents.devimport { c, useProp } from "atomico";
const style = /*css*/ `
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`;
function myCounter() {
let [count, setCount] = useProp("count");
return (
<host shadowDom>
<style>{style}</style>
<button onclick={() => setCount(count - 1)}>-</button>
<span>{count}</span>
<button onclick={() => setCount(count + 1)}>+</button>
</host>
);
}
myCounter.props = {
count: {
type: Number,
reflect: true,
value: 0,
},
};
customElements.define("my-counter", c(myCounter));
Dependencies
atomico : ^1.45.1
Component size including library
Minified - Uncompressed | 8,746 |
Minified + Gzip | 4,049 |
Minified + Brotli | 3,649 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
{
"presets": [
[
"@babel/preset-react",
{
"throwIfNamespace": false,
"runtime": "automatic",
"importSource": "atomico"
}
]
]
}
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
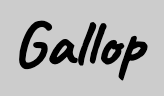
Gallop
Use full power of web components
GitHub: https://github.com/tarnishablec/gallop
Try in WebComponents.devimport { component, css, useState, useStyle, html } from "@gallop/gallop";
component("my-counter", () => {
const [state] = useState({ count: 0 });
useStyle(
() => css`
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`,
[]
);
return html`
<button @click="${() => state.count--}">
-
</button>
<span>${state.count}</span>
<button @click="${() => state.count++}">
+
</button>
`;
});
Dependencies
@gallop/gallop : ^1.0.13
Component size including library
Minified - Uncompressed | 11,470 |
Minified + Gzip | 4,344 |
Minified + Brotli | 3,887 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Haunted
React's Hooks API implemented for web components 👻
GitHub: https://github.com/matthewp/haunted
Try in WebComponents.devimport { html, component, useState } from "haunted";
function Counter() {
const [count, setCount] = useState(0);
return html`
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button @click=${() => setCount(count - 1)}>
-
</button>
<span>${count}</span>
<button @click=${() => setCount(count + 1)}>
+
</button>
`;
}
customElements.define("my-counter", component(Counter));
Dependencies
haunted : ^4.8.3
Component size including library
Minified - Uncompressed | 16,303 |
Minified + Gzip | 5,547 |
Minified + Brotli | 4,977 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Heresy w/Hook
Don't simulate the DOM. Be the DOM. React-like Custom Elements via the V1 API built-in extends.
Homepage: https://medium.com/@WebReflection/any-holy-grail-for-web-components-c3d4973f3f3f
GitHub: https://github.com/WebReflection/heresy
Try in WebComponents.devimport { define } from "heresy";
define("MyCounter", {
style: (MyCounter) => `
${MyCounter} * {
font-size: 200%;
}
${MyCounter} span {
width: 4rem;
display: inline-block;
text-align: center;
}
${MyCounter} button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`,
render({ useState }) {
const [count, update] = useState(0);
this.html`
<button onclick="${() => update(count - 1)}">-</button>
<span>${count}</span>
<button onclick="${() => update(count + 1)}">+</button>
`;
},
});
Dependencies
heresy : ^1.0.4
Component size including library
Minified - Uncompressed | 21,531 |
Minified + Gzip | 8,808 |
Minified + Brotli | 8,013 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Ficus w/ lit-html
Lightweight functions for developing applications using web components
Homepage: https://docs.ficusjs.org/
GitHub: https://github.com/ficusjs/ficusjs
Try in WebComponents.devimport { render as renderer, html } from "lit-html";
import { createComponent } from "ficusjs";
createComponent("my-counter", {
root: "shadow",
state() {
return { count: 0 };
},
renderer,
inc() {
this.state.count++;
},
dec() {
this.state.count--;
},
render() {
return html`
<style>
span,
button {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<div>
<button @click="${this.dec}">-</button>
<span>${this.state.count}</span>
<button @click="${this.inc}">+</button>
</div>
`;
},
});
Dependencies
ficusjs : ^3.15.0
lit-html : ^2.1.3
Component size including library
Minified - Uncompressed | 14,515 |
Minified + Gzip | 5,416 |
Minified + Brotli | 4,858 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Heresy
Don't simulate the DOM. Be the DOM. React-like Custom Elements via the V1 API built-in extends.
Homepage: https://medium.com/@WebReflection/any-holy-grail-for-web-components-c3d4973f3f3f
GitHub: https://github.com/WebReflection/heresy
Try in WebComponents.devimport { define } from "heresy";
define("MyCounter", {
style: (MyCounter) => `
${MyCounter} * {
font-size: 200%;
}
${MyCounter} span {
width: 4rem;
display: inline-block;
text-align: center;
}
${MyCounter} button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`,
oninit() {
this.count = 0;
},
onclick({ currentTarget }) {
this[currentTarget.dataset.op]();
this.render();
},
inc() {
this.count++;
},
dec() {
this.count--;
},
render() {
this.html`
<button data-op="dec" onclick="${this}">-</button>
<span>${this.count}</span>
<button data-op="inc" onclick="${this}">+</button>
`;
},
});
Dependencies
heresy : ^1.0.4
Component size including library
Minified - Uncompressed | 21,654 |
Minified + Gzip | 8,847 |
Minified + Brotli | 8,037 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
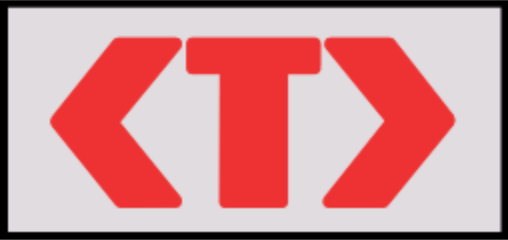
htmlhammer
Write HTML with JavaScript using real HTML tag names.
GitHub: https://github.com/vsmid/htmlhammer
Try in WebComponents.devimport { style, button, span, customElement } from 'htmlhammer';
const ButtonStyle = {
fontSize: "200%",
width: "4rem",
height: "4rem",
border: "none",
borderRadius: "10px",
backgroundColor: "seagreen",
color: "white"
};
const SpanStyle = {
fontSize: "200%",
width: "4rem",
display: "inline-block",
textAlign: "center"
}
const MyCounter = customElement("my-counter", {
Count: 0,
CounterDisplay: null,
connectedCallback() {
this.attachShadow({mode: "open"})
this.shadowRoot.append(
button({ id: "dec", onclick: this.Dec, style: ButtonStyle }, "-"),
this.CounterDisplay = span({ style: SpanStyle }, this.Count),
button({ id: "inc", onclick: this.Inc, style: ButtonStyle }, "+")
);
},
Inc() {
this.Update(++this.Count);
},
Dec() {
this.Update(--this.Count);
},
Update(count) {
this.CounterDisplay.textContent = count;
}
});
Dependencies
htmlhammer : ^3.5.2
Component size including library
Minified - Uncompressed | 5,878 |
Minified + Gzip | 2,761 |
Minified + Brotli | 2,346 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Hybrids
Hybrids is a UI library for creating web components with strong declarative and functional approach based on plain objects and pure functions.
Homepage: https://hybrids.js.org
GitHub: https://github.com/hybridsjs/hybrids
Try in WebComponents.devimport { html, define } from "hybrids";
function inc(host) {
host.count++;
}
function dec(host) {
host.count--;
}
const MyCounter = {
tag: "my-counter",
count: 0,
render: ({ count }) => html`
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button onclick="${dec}">-</button>
<span>${count}</span>
<button onclick="${inc}">+</button>
`,
};
define(MyCounter);
Dependencies
hybrids : ^7.0.5
Component size including library
Minified - Uncompressed | 17,003 |
Minified + Gzip | 6,550 |
Minified + Brotli | 5,910 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Kirei
An in browser implementation of Vue 3 (limited to Composition API)
GitHub: https://github.com/ifaxity/kirei
Try in WebComponents.devimport { defineComponent, html, css, ref } from '@kirei/element/dist/element.module.js';
defineComponent({
name: 'MyCounter',
styles: css`
span, button {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
`,
setup() {
const counter = ref(0);
function dec() {
counter.value -= 1;
}
function inc() {
counter.value += 1;
}
return () => html`
<button @click=${dec}>-</button>
<span>${counter.value}</span>
<button @click=${inc}>+</button>
`;
}
});
Dependencies
@kirei/element : ^2.0.4
mobx : ^5.15.4
Component size including library
Minified - Uncompressed | 29,813 |
Minified + Gzip | 11,654 |
Minified + Brotli | 10,541 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
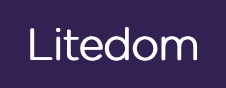
Litedom
A very small (3kb) view library that is packed: Web Components, Custom Element, Template Literals, Reactive, Data Binding, One Way Data Flow, Two-way data binding, Event Handling, Props, Lifecycle, State Management, Computed Properties, Directives. No dependencies, no virtual dom, no build tool. Wow! ...but it's a just a view library!
Homepage: https://litedom.js.org/
GitHub: https://github.com/mardix/litedom
Try in WebComponents.devimport Litedom from "litedom";
Litedom({
tagName: "my-counter",
shadowDOM: true,
template: `
<button @click="dec">-</button>
<span>{this.count}</span>
<button @click="inc">+</button>
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
`,
data: {
count: 0,
},
dec() {
this.data.count--;
},
inc() {
this.data.count++;
},
});
Dependencies
litedom : ^0.12.1
Component size including library
Minified - Uncompressed | 9,502 |
Minified + Gzip | 3,870 |
Minified + Brotli | 3,428 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...

Ottavino
Tiny, Fast and Declarative User Interface Development. Using native custom elements API (but not only). As simple as it gets.
GitHub: https://github.com/betterthancode/ottavino
Try in WebComponents.devimport { component } from "ottavino";
component({
tag: "my-counter",
shadow: true,
template: `
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button onclick="{{this.decrease()}}">-</button>
<span>{{this.count}}</span>
<button onclick="{{this.increase()}}" >+</button>
`,
properties: {
count: 0,
},
this: {
increase: function () {
this.count++;
},
decrease: function () {
this.count--;
},
},
});
Dependencies
ottavino : ^0.2.4
Component size including library
Minified - Uncompressed | 4,476 |
Minified + Gzip | 1,992 |
Minified + Brotli | 1,726 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Swiss
Functional custom elements
GitHub: https://github.com/luwes/swiss
Try in WebComponents.devimport { define } from "swiss";
import { html, render } from "lit-html";
const Counter = (CE) => (el) => {
el.attachShadow({ mode: "open" });
return {
update: () =>
render(
html`
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button @click="${() => el.count--}">-</button>
<span>${el.count}</span>
<button @click="${() => el.count++}">+</button>
`,
el.shadowRoot
),
};
};
define("my-counter", {
props: { count: 0 },
setup: Counter,
});
Dependencies
swiss : ^2.4.0
Component size including library
Minified - Uncompressed | 11,362 |
Minified + Gzip | 4,572 |
Minified + Brotli | 4,123 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
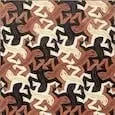
Synergy
Synergy.js is a 4kB runtime library that gives you everything you need to define Web Components using standard HTML, JavaScript and CSS.
Homepage: https://synergyjs.org/
GitHub: https://github.com/defx/synergy
Try in WebComponents.devimport { define } from 'synergy';
const style = /*css*/`
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
const template = /*html*/`
<style>${style}</style>
<button :onclick="dec()">
-
</button>
<span>{{ count }}</span>
<button :onclick="inc()">
+
</button>`;
const model = () => ({
count: 0,
inc() {
this.count += 1;
},
dec() {
this.count -= 1;
}
});
define("my-counter", model, template, { shadow: 'open' });
Dependencies
synergy : ^7.0.3
Component size including library
Minified - Uncompressed | 10,499 |
Minified + Gzip | 4,191 |
Minified + Brotli | 3,724 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
uce
µhtml based Custom Elements.
Homepage: https://github.com/WebReflection/uce
GitHub: https://github.com/WebReflection/uce
Try in WebComponents.devimport "uce";
const MyCounter = ({ define }) => {
define("my-counter", {
attachShadow: { mode: "open" },
props: { count: 0 },
bound: ["inc", "dec"],
inc() {
this.count++;
this.render();
},
dec() {
this.count--;
this.render();
},
render() {
this.html`
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button onclick="${this.dec}">-</button>
<span>${this.count}</span>
<button onclick="${this.inc}">+</button>
`;
},
});
};
customElements
.whenDefined("uce-lib")
.then(() => MyCounter(customElements.get("uce-lib")));
Dependencies
uce : ^1.16.5
Component size including library
Minified - Uncompressed | 10,533 |
Minified + Gzip | 4,644 |
Minified + Brotli | 4,202 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
twind
Homepage: https://twind.dev/
GitHub: https://github.com/tw-in-js/twind
Try in WebComponents.devimport { create, cssomSheet } from 'twind'
// 1. Create separate CSSStyleSheet
const sheet = cssomSheet({ target: new CSSStyleSheet() })
// 2. Use that to create an own twind instance
const { tw } = create({ sheet })
const template = document.createElement("template");
template.innerHTML = /*html*/`
<p class="${tw`invisible`}">This uses CSSStyleSheet but<br>it's not supported in Firefox.</p>
<button class="${tw`rounded-lg px-7 py-4 text(white 3xl) bg-green-600`}" id="dec">-</button>
<span class="${tw`inline-block w-14 text(3xl center)`}" id="count"></span>
<button class="${tw`rounded-lg px-7 py-4 text(white 3xl) bg-green-600`}" id="inc">+</button>`;
class MyCounter extends HTMLElement {
constructor() {
super();
this.count = 0;
this.attachShadow({ mode: "open" });
this.shadowRoot.adoptedStyleSheets = [sheet.target];
}
connectedCallback() {
this.shadowRoot.appendChild(template.content.cloneNode(true));
this.shadowRoot.getElementById("inc").onclick = () => this.inc();
this.shadowRoot.getElementById("dec").onclick = () => this.dec();
this.update(this.count);
}
inc() {
this.update(++this.count);
}
dec() {
this.update(--this.count);
}
update(count) {
this.shadowRoot.getElementById("count").innerHTML = count;
}
}
customElements.define("my-counter", MyCounter);
Dependencies
twind : ^0.16.16
Component size including library
Minified - Uncompressed | 35,619 |
Minified + Gzip | 14,205 |
Minified + Brotli | 12,687 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
twind + LitElement
twind - A small compiler that turns Tailwind short hand into CSS rules at run, build or serve time. If you have used Tailwind and/or a CSS-in-JS solution before then most of the API will feel very familiar.
Homepage: https://twind.dev/
GitHub: https://github.com/tw-in-js/twind
Try in WebComponents.devimport { LitElement, html } from "lit";
import { create, cssomSheet } from 'twind'
// 1. Create separate CSSStyleSheet
const sheet = cssomSheet({ target: new CSSStyleSheet() })
// 2. Use that to create an own twind instance
const { tw } = create({ sheet })
export class MyCounter extends LitElement {
static properties = {
count: { type: Number },
};
static styles = [sheet.target];
constructor() {
super();
this.count = 0;
}
inc() {
this.count++;
}
dec() {
this.count--;
}
render() {
return html`
<p class="${tw`invisible`}">This uses CSSStyleSheet but<br>it's not supported in Firefox.</p>
<button class="${tw`rounded-lg px-7 py-4 text(white 3xl) bg-green-600`}" @click="${this.dec}">-</button>
<span class="${tw`inline-block w-14 text(3xl center)`}">${this.count}</span>
<button class="${tw`rounded-lg px-7 py-4 text(white 3xl) bg-green-600`}" @click="${this.inc}">+</button>
`;
}
}
customElements.define("my-counter", MyCounter);
Dependencies
twind : ^0.16.16
lit-element : ^3.1.2
Component size including library
Minified - Uncompressed | 51,177 |
Minified + Gzip | 19,449 |
Minified + Brotli | 17,585 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
{
"plugins": [
[
"@babel/plugin-proposal-decorators",
{
"decoratorsBeforeExport": true
}
],
"@babel/plugin-proposal-class-properties"
]
}
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Lightning Web Components
⚡️ LWC - A Blazing Fast, Enterprise-Grade Web Components Foundation
Homepage: https://lwc.dev
GitHub: https://github.com/salesforce/lwc
Try in WebComponents.devimport { LightningElement } from "lwc";
export default class MyCounter extends LightningElement {
count = 0;
inc() {
this.count++;
}
dec() {
this.count--;
}
}
customElements.define("my-counter", MyCounter.CustomElementConstructor);
Dependencies
lwc : 2.4.0
Component size including library
Minified - Uncompressed | 42,811 |
Minified + Gzip | 14,273 |
Minified + Brotli | 12,777 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following LWC settings:
const outputConfig: {
format: 'esm',
sourcemap: true,
};
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Lume Element
Create Custom Elements with reactivity and automatic re-rendering
GitHub: https://github.com/lume/element
Try in WebComponents.devimport {Element, reactify} from '@lume/element'
import {html} from '@lume/element/dist/html'
export class MyCounter extends Element {
count = 0;
constructor() {
super()
reactify(this, ['count'])
}
template = () => html`
<button onclick=${() => (this.count -= 1)}>-</button>
<span>${() => this.count}</span>
<button onclick=${() => (this.count += 1)}>+</button>
`
css = /*css*/ `
* { font-size: 200%; }
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem; height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
outline: none;
cursor: pointer;
}
`
}
customElements.define('my-counter', MyCounter)
Dependencies
@lume/element : ^0.5.7
Component size including library
Minified - Uncompressed | 37,362 |
Minified + Gzip | 13,123 |
Minified + Brotli | 11,918 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with thesolid
preset:
{
"presets": [
"solid"
]
}
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Prism
Prism is a experimental compiler for building isomorphic web applications with web components.
GitHub: https://github.com/kaleidawave/prism
Try in WebComponents.dev<template>
<button @click="dec">
-
</button>
<span>{count}</span>
<button @click="inc">
+
</button>
</template>
<script>
@Default({count: 0})
class MyCounter extends Component<{count: number}> {
inc() {
this.data.count++;
}
dec() {
this.data.count--;
}
}
</script>
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
Dependencies
@kaleidawave/prism :
Component size including library
Minified - Uncompressed | 1,894 |
Minified + Gzip | 979 |
Minified + Brotli | 811 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Solid Element
The Deceptively Simple User Interface Library
GitHub: https://github.com/ryansolid/solid
Try in WebComponents.devimport { createSignal } from "solid-js";
import { customElement } from "solid-element";
const style = `div * {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
customElement("my-counter", () => {
const [count, setCount] = createSignal(0);
return (
<div>
<style>{style}</style>
<button onClick={() => setCount(count() - 1)}>-</button>
<span>{count}</span>
<button onClick={() => setCount(count() + 1)}>+</button>
</div>
);
});
Dependencies
solid-js : ^1.3.7
solid-element : ^1.3.7
Component size including library
Minified - Uncompressed | 11,016 |
Minified + Gzip | 4,177 |
Minified + Brotli | 3,743 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with thesolid
preset:
{
"presets": [
"solid"
]
}
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Stencil
Stencil is a toolchain for building reusable, scalable Design Systems. Generate small, blazing fast, and 100% standards based Web Components that run in every browser.
Homepage: https://stenciljs.com/
GitHub: https://github.com/ionic-team/stencil
Try in WebComponents.dev/* @jsx h */
import { Component, Prop, h, Host } from '@stencil/core';
@Component({
tag: "my-counter",
styleUrl: "index.css",
shadow: true,
})
export class MyCounter {
@Prop() count: number = 0;
inc() {
this.count++;
}
dec() {
this.count--;
}
render() {
return (
<Host>
<button onClick={this.dec.bind(this)}>-</button>
<span>{this.count}</span>
<button onClick={this.inc.bind(this)}>+</button>
</Host>
);
}
}
Dependencies
@stencil/core : ^2.13.0
Component size including library
Minified - Uncompressed | 14,615 |
Minified + Gzip | 6,144 |
Minified + Brotli | 5,527 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been compiled with Stencil CLI and settings:
// stencil.tsconfig.js
...
"compilerOptions": {
"allowSyntheticDefaultImports": true,
"allowUnreachableCode": false,
"declaration": false,
"experimentalDecorators": true,
"lib": ["DOM", "ES2018"],
"moduleResolution": "node",
"module": "esnext",
"target": "es2017",
"noUnusedLocals": true,
"noUnusedParameters": true,
"jsx": "react",
"jsxFactory": "h",
"types": []
}
...
//stencil.config.js
import { Config } from '@stencil/core';
export const config: Config = {
srcDir: 'stencil/src',
namespace: 'my-counter',
tsconfig: 'stencil.tsconfig.json',
hashFileNames: false,
plugins: [],
outputTargets: [
{
type: 'dist-custom-elements-bundle',
},
],
hydratedFlag: null,
extras: {
cssVarsShim: false,
dynamicImportShim: false,
safari10: false,
scriptDataOpts: false,
shadowDomShim: false,
},
};
Bundling configuration
See details
Bundled by Stencil CLISvelte (with {customElement: true})
Cybernetically enhanced web apps
Homepage: https://svelte.dev/
GitHub: https://github.com/sveltejs/svelte
Try in WebComponents.dev<svelte:options tag="my-counter" />
<script>
let count = 0;
function inc() {
count++;
}
function dec() {
count--;
}
</script>
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button on:click={dec}>
-
</button>
<span>{count}</span>
<button on:click={inc}>
+
</button>
Dependencies
svelte : ^3.46.4
Component size including library
Minified - Uncompressed | 4,070 |
Minified + Gzip | 1,918 |
Minified + Brotli | 1,685 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Svelte settings:
const options = {
format: 'esm',
customElement: true
};
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Preact w/Class (wrapped with preact-custom-element)
Fast 3kB alternative to React with the same modern API.
Homepage: https://preactjs.com/
GitHub: https://github.com/preactjs/preact
Try in WebComponents.devimport { createCustomElement } from "@wcd/preact-custom-element";
import { Component, html } from "htm/preact";
import "preact";
class MyCounter extends Component {
state = {
count: 0,
};
inc = () => {
this.setState((prev) => ({ count: prev.count + 1 }));
};
dec = () => {
this.setState((prev) => ({ count: prev.count - 1 }));
};
render(props, state) {
return html`
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button onClick=${this.dec}>
-
</button>
<span>${state.count}</span>
<button onClick=${this.inc}>
+
</button>
`;
}
}
customElements.define("my-counter", createCustomElement(MyCounter, ["count"]));
Dependencies
preact : ^10.6.6
@wcd/preact-custom-element : ^3.1.3
Component size including library
Minified - Uncompressed | 12,037 |
Minified + Gzip | 4,903 |
Minified + Brotli | 4,481 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Babel settings:
[
[
Babel.availablePresets['env'],
{
targets: {
browsers:
'last 2 Chrome versions, last 2 Firefox versions, last 2 Safari versions, last 2 iOS versions, last 2 Android versions',
},
modules: 'false',
bugfixes: true,
},
],
[Babel.availablePresets['react']],
],
plugins: [
[Babel.availablePlugins['proposal-decorators'], { legacy: true }],
[Babel.availablePlugins['proposal-class-properties'], { loose: true }],
]
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
React w/Class (wrapped with react-to-webcomponent)
A JavaScript library for building user interfaces
Homepage: https://reactjs.org/
GitHub: https://github.com/facebook/react/
Try in WebComponents.devimport React from "react";
import ReactDOM from "react-dom";
import reactToWebComponent from "react-to-webcomponent";
interface State {
count: number;
}
interface Props {}
export default class MyCounter extends React.Component<Props, State> {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
render() {
const styles = `.my-counter * {
font-size: 200%;
}
.my-counter span {
width: 4rem;
display: inline-block;
text-align: center;
}
.my-counter button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
return (
<div className="my-counter">
<style>{styles}</style>
<button onClick={() => this.setState({ count: this.state.count - 1 })}>
-
</button>
<span>{this.state.count}</span>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>
+
</button>
</div>
);
}
}
customElements.define(
"my-counter",
reactToWebComponent(MyCounter, React, ReactDOM)
);
Dependencies
react : ^17.0.2
react-dom : ^17.0.2
react-to-webcomponent : ^1.5.1
Component size including library
Minified - Uncompressed | 131,660 |
Minified + Gzip | 42,508 |
Minified + Brotli | 37,428 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following TypeScript settings: {
module: ts.ModuleKind.ESNext,
experimentalDecorators: true,
emitDecoratorMetadata: true,
importHelpers: true,
lib: ['ESNext', 'dom'],
target: ts.ScriptTarget.ESNext
};
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
React w/Hook (wrapped with react-to-webcomponent)
A JavaScript library for building user interfaces
Homepage: https://reactjs.org/
GitHub: https://github.com/facebook/react/
Try in WebComponents.devimport React, { useState } from "react";
import ReactDOM from "react-dom";
import reactToWebComponent from "react-to-webcomponent";
export default function MyCounter() {
const [count, setCount] = useState(0);
const styles = `
.my-counter * {
font-size: 200%;
}
.my-counter span {
width: 4rem;
display: inline-block;
text-align: center;
}
.my-counter button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}`;
return (
<div className="my-counter">
<style>{styles}</style>
<button onClick={() => setCount(count - 1)}>-</button>
<span>{count}</span>
<button onClick={() => setCount(count + 1)}>+</button>
</div>
);
}
customElements.define(
"my-counter",
reactToWebComponent(MyCounter, React, ReactDOM)
);
Dependencies
react : ^17.0.2
react-dom : ^17.0.2
react-to-webcomponent : ^1.5.1
Component size including library
Minified - Uncompressed | 131,515 |
Minified + Gzip | 42,475 |
Minified + Brotli | 37,408 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following TypeScript settings: {
module: ts.ModuleKind.ESNext,
experimentalDecorators: true,
emitDecoratorMetadata: true,
importHelpers: true,
lib: ['ESNext', 'dom'],
target: ts.ScriptTarget.ESNext
};
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Riot (wrapped with @riotjs/custom-elements)
Simple and elegant component-based UI library
Homepage: https://riot.js.org/
GitHub: https://github.com/riot/riot
Try in WebComponents.dev<my-component>
<style>
* {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 64px;
height: 64px;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
<button onclick={dec}>
-
</button>
<span>{state.count}</span>
<button onclick={inc}>
+
</button>
<script>
export default {
onBeforeMount(props, state) {
this.state = {
count: 0
}
},
inc() {
this.update({
count: this.state.count+1
})
},
dec() {
this.update({
count: this.state.count-1
})
},
}
</script>
</my-component>
Dependencies
riot : ^6.1.2
@riotjs/custom-elements : ^6.0.0
Component size including library
Minified - Uncompressed | 16,857 |
Minified + Gzip | 6,104 |
Minified + Brotli | 5,480 |
Composition
Open Bundle VisualizerCompilation configuration
See details
All Sources have been Transpiled by WebComponents.dev compiler with the following Riot settings:
const settings = {
scopedCss: false,
};
Bundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Vue2 (wrapped with vue-custom-element)
🖖 Vue.js is a progressive, incrementally-adoptable JavaScript framework for building UI on the web.
Homepage: https://vuejs.org/
GitHub: https://github.com/vuejs/vue
Try in WebComponents.dev<template>
<div>
<button @click="this.dec">
-
</button>
<span>{{count}}</span>
<button @click="this.inc">
+
</button>
</div>
</template>
<script>
export default {
tag: 'my-counter',
name: 'MyCounter',
data() {
return { count: 0 }
},
methods: {
inc: function() {
this.count++;
},
dec: function() {
this.count--;
}
}
};
</script>
Dependencies
vue : ^3.2.31
@vue/web-component-wrapper : ^1.3.0
Component size including library
Minified - Uncompressed | 70,007 |
Minified + Gzip | 25,151 |
Minified + Brotli | 22,658 |
Composition
Open Bundle VisualizerCompilation configuration
See details
Vue sources have been Transpiled by rollup-plugin-vueBundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Vue3 (wrapped within a minimum Web Component layer)
🖖 Vue.js is a progressive, incrementally-adoptable JavaScript framework for building UI on the web.
Homepage: https://vuejs.org/
GitHub: https://github.com/vuejs/vue
Try in WebComponents.dev<template>
<div>
<button @click="dec">
-
</button>
<span>{{ state.count }}</span>
<button @click="inc">
+
</button>
</div>
</template>
<script>
import { reactive } from "vue";
export default {
setup() {
const state = reactive({
count: 0,
});
function inc() {
state.count++;
}
function dec() {
state.count--;
}
return {
state,
inc,
dec,
};
},
};
</script>
<style scoped>
span,
button {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
Dependencies
vue@next :
Component size including library
Minified - Uncompressed | 51,371 |
Minified + Gzip | 20,154 |
Minified + Brotli | 18,296 |
Composition
Open Bundle VisualizerCompilation configuration
See details
Vue sources have been Transpiled by rollup-plugin-vueBundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Vue3 CE (`defineCustomElement` +v3.2)
🖖 Vue.js is a progressive, incrementally-adoptable JavaScript framework for building UI on the web.
Homepage: https://vuejs.org/
GitHub: https://github.com/vuejs/vue
Try in WebComponents.dev<template>
<div>
<button @click="dec">
-
</button>
<span>{{ state.count }}</span>
<button @click="inc">
+
</button>
</div>
</template>
<script>
import { reactive } from "vue";
export default {
setup() {
const state = reactive({
count: 0,
});
function inc() {
state.count++;
}
function dec() {
state.count--;
}
return {
state,
inc,
dec,
};
},
};
</script>
<style scoped>
span,
button {
font-size: 200%;
}
span {
width: 4rem;
display: inline-block;
text-align: center;
}
button {
width: 4rem;
height: 4rem;
border: none;
border-radius: 10px;
background-color: seagreen;
color: white;
}
</style>
Dependencies
vue@next :
Component size including library
Minified - Uncompressed | 53,087 |
Minified + Gzip | 20,684 |
Minified + Brotli | 18,809 |
Composition
Open Bundle VisualizerCompilation configuration
See details
Vue sources have been Transpiled by rollup-plugin-vueBundling configuration
See details
Bundled with Rollup
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
import replace from '@rollup/plugin-replace';
import { terser } from 'rollup-plugin-terser';
...
input: 'source.js',
output: {
dir: 'dist'),
format: 'esm',
},
plugins: [
replace({
'process.env.NODE_ENV': JSON.stringify('production'),
}),
withdeps ? resolve() : undefined,
commonjs( /* { namedExports: for React } */ ),
terser({ output: { comments: false } }),
...
Bundle Size Comparison
Bundle size of 1 component
If you only deliver a single <my-counter/>
you have the full cost of the library for a single component.
This is the total cost of a single component with the library included.
Notes
The bare HTMLElement
component doesn't have any dependency and creates a unmatched tiny package.
Prism
is still the smallest package for a single component, keeping its 2nd place, but the Readymade
flavor is now really not far behind it! Really well work done by their teams, congrats!
The rest of the pool still remains the same, with very few different changes, with minor updates here and there, leading to reducing or increasing of few KB all of the bundles.
However, a special mention to elemx that cut its total cost by about 50%! Even if coming with mobx
for the reactivity leads to an increased bundle size. Maybe the next edition will be an occasion to try to replace it with lobx
, a lighter implementation.
On the overview, we still go to the same conclusion: complex reactive frameworks like React or Vue comes well in the WebComponents area if you know them already, but aren't the best choice in term in footprint. You may prefer to use raw HTMLElement
variants, or stay focused on dedicated, lightweight libraries.
Caution
Some libraries bring significant value and are more feature rich than others. It's normal to see larger sizes in these cases. Nevertheless, for a simple counter component, we would like to see more tree-shaking happening.
On the other hand, once you start having multiple components using a broad spectrum of the library capabilities, you end up with the entire library anyway.
Estimated Bundle size of 30 components using the same library
This is an estimated size of a bundle of 30 <my-counter />
-like components using the same library. All components will share the library code so the estimated size is calculated with: 1 bundle-with-dependencies + 29x components-without-dependencies.
Notes
We still see that some libraries managed to be smaller than bare HTMLElement
, thanks to the compression algorithms allowing to significantly shrink the overall bundle size. Brotli is doing an impressive work on this part.
An interesting aspect is that by choosing not to share logics between components like Prism does, no tree shaking can be involved, resulting into an increasing bundle size in comparison to others. Making the choice of using a set of commons, and performing a proper tree shaking, often leads to better results.
Performance
Performance of Parsing JavaScript + Create DOM tree
Benchmarked with Tachometer on Google Chrome Version 98.0.4758.102 (Official Build) (arm64).
The benchmark page is composed of 50 <my-counter /my-counter>
laid out in a single page with the library bundle code inlined.
Notes
Take into consideration that there is a margin of error of 2ms.
Everything runs locally so the network download time is not taken into account here.
Everything runs on a relatively powerful laptop and results could be completely different on a lower-end device like a phone or a tablet.
Things that play a role in this performance figure are:
- The size of the JavaScript to parse (more JavaScript = longer).
- The performance of the library to create the DOM nodes from scratch.
Final Notes
It's hard to find so much diversity in a technical solution. It tells a lot about Web Components and the low level API provided. All the 61 variants could be on the same page if we wanted to!
On bundle size, the results are already very promising but there is still room for improvement:
- Some libraries would benefit from splitting their features, to better benefit from tree-shaking.
- Except Stencil and Svelte, none of the libraries offer CSS minification out of the box.
Web Components are well supported in browsers now. Still, for old browsers (including IE11), polyfills are required. The bundle sizes reported here are only for modern browsers that don't need any polyfills. It was too much additional work to include polyfills in this post. Maybe later... before they become obsolete.
We will report on new libraries and updates. Make sure to follow us on Twitter.
Feedback or Questions
Chat with us on our Discord or Twitter channels.
Thanks
Thanks to all those who helped us review and correct this analysis.
- @Uppercod creator of Atomico
- @adamdbradley creator of Stencil
- @matthewcp creator of Haunted
- @eavichay creator of SlimJs, Ottavino and Neow (in alpha)
- @WebReflection creator of Heresy, HyperHTML, lighterhtml, uhtml, uce and uce-template
- @smalluban creator of Hybrids
- @_developit creator of Preact
- @KevinJHill @diervo @pmdartus of the LWC team
- @Rich_Harris representing Svelte
- @justinfagnani from lit-html and LitElement team
- @RyanCarniato creator of Solid
- @trusktr creator of @lume/element
- @luwes creator of Swiss
- @Tarnishablec creator of Gallop
- @EisenbergEffect on team FAST
- @dee_bloo creator of Joist
- @iplayitofflegit creator of Readymade
- @ducksoupdev creator of Ficus JS
- @yysun creator of AppRun
- @keithamus contributor on jtml & Catalyst
- @v_smid creator of htmlhammer
- @jleesons creator of Exalt
- @mattdonkin & @davide_v creator of Synergy
- @kaleidawave creator of Prism compiler
- @lamplightdev creator of Wafer
- @bebraw creator of Sidewind
- @jogibear9988 creator of base-custom-webcomponent
- @woutervroege creator of html-element-property-mixins
- Tabs from @lion/tabs - Thanks @dakmor and ING
- Charts library by Charts.js
Changes
since Nov 2021 release
- All libraries upgraded to their last versions.
- Due to compilation issue, we're stuck to
lwc@2.4.0
compiler for Lightning Web Components. We're focusing on fixing it for the next release. - Added libraries as a new variants:
node-projects/base-custom-webcomponent
html-element-property-mixins
- Added in Performance chart:
- Stencil
- Haunted
- Htmlhammer
- Github's Catalyst
since May 2021 release
- All libraries upgraded to minor versions
- Libraries upgraded to major versions:
- Apprun
- Exalt
- Gallop
- LWC
- Slimjs
- Added incremental-dom
- Added Sidewind
- Added Vue +3.2 CE
- Added Wafer
- Re-adding CanJS
- Removed Angular - Build issues with version 13
since January 2021 release
- All libraries upgraded to @latest
- Added htmlhammer
- Added Exalt
- Added Synergy
- Added Prism compiler
- Upgraded lit next-major to the new Lit (v2.0.0rc)
- Updated uce-template counter with .uce
- Updated Atomico counter
- Updated Readymade counter
- Removed CanJS - compilation issues
Brought to you by Twitter Discord